Apps Script Course: Dialog Boxes
Dialog boxes are used to display information, ask for user confirmation, or to prompt the user to enter information.
Alert
The alert method is used to display a dialog box with different possible parameters.
With a single argument, you simply display a message with an OK button:
SpreadsheetApp.getUi().alert('Deletion confirmed');
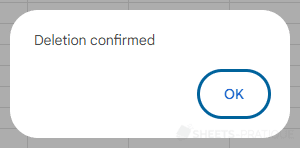
If you enter a second argument, it defines which buttons to display.
The possible choices are:
Constant | Buttons |
---|---|
OK | OK |
OK_CANCEL | OK, Cancel |
YES_NO | Yes, No |
YES_NO_CANCEL | Yes, No, Cancel |
For example:
const ui = SpreadsheetApp.getUi();
ui.alert('Confirm deletion?', ui.ButtonSet.YES_NO);
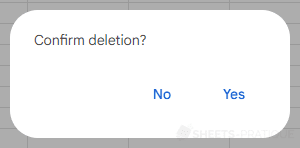
If you enter three arguments, the first will be the title, the second the text, and the third the buttons:
const ui = SpreadsheetApp.getUi();
ui.alert('Confirmation Request', 'Confirm deletion?', ui.ButtonSet.YES_NO_CANCEL);
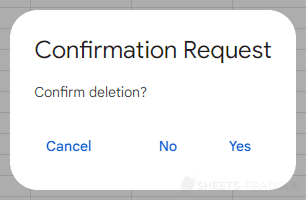
To know the user's choice and whether or not to take an action, you need to retrieve the value returned by alert:
const ui = SpreadsheetApp.getUi();
const choice = ui.alert('Confirm deletion?', ui.ButtonSet.YES_NO);
Then compare the returned value:
Constant | User Choice |
---|---|
OK | OK Button |
CANCEL | Cancel Button |
YES | Yes Button |
NO | No Button |
CLOSE | Dialog Box Close Button |
For example:
const ui = SpreadsheetApp.getUi();
const choice = ui.alert('Confirm deletion?', ui.ButtonSet.YES_NO);
// If the user clicks Yes
if (choice == ui.Button.YES) {
// Display another dialog box
ui.alert('Deletion confirmed!');
}
Or:
const ui = SpreadsheetApp.getUi();
// If the user clicks Yes
if (ui.alert('Confirm deletion?', ui.ButtonSet.YES_NO) == ui.Button.YES) {
// Display another dialog box
ui.alert('Deletion confirmed!');
}
Toast
The toast method displays a small window at the bottom right of the sheet that disappears after a few seconds.
It can be used for example to display a confirmation (which requires no action on the part of the user to close):
SpreadsheetApp.getActive().toast('Deletion confirmed!');
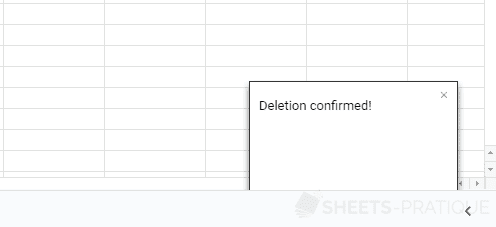
It is possible to add a title as a second argument:
SpreadsheetApp.getActive().toast('All data has been deleted.', 'Deletion confirmed');
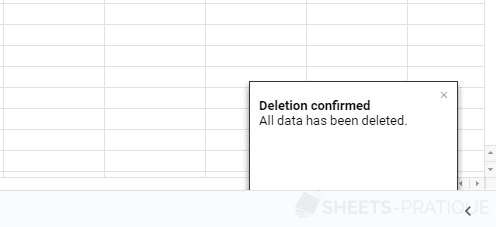
You can also specify the duration (in seconds) as a third argument:
SpreadsheetApp.getActive().toast('OK', null, 1.5);
Prompt
The prompt method displays a dialog box where the user is prompted to enter information.
Just like the alert method, you can enter from 1 to 3 arguments.
Example with a single argument:
const response = SpreadsheetApp.getUi().prompt('Enter your name:');
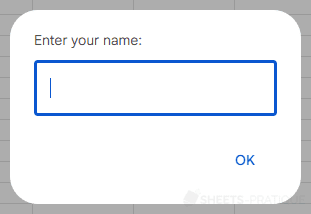
A second argument allows you to choose the buttons (you have the same button choices as with alert):
const ui = SpreadsheetApp.getUi();
const response = ui.prompt('Enter your name and click OK:', ui.ButtonSet.OK_CANCEL);
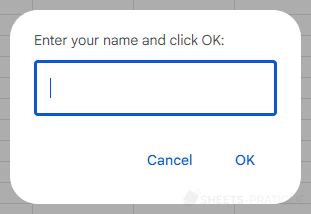
And finally, an example with 3 arguments (title, text, buttons):
const ui = SpreadsheetApp.getUi();
const response = ui.prompt('Registration', 'Enter your name and click OK:', ui.ButtonSet.OK_CANCEL);
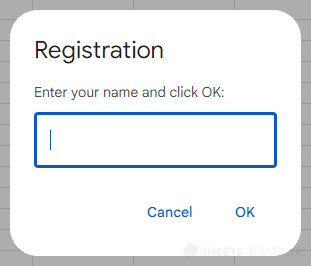
From the value returned by prompt, you can retrieve both the entered value and the clicked button:
const ui = SpreadsheetApp.getUi();
const response = ui.prompt('Registration', 'Enter your name and click OK:', ui.ButtonSet.OK_CANCEL);
if (response.getSelectedButton() == ui.Button.OK) {
ui.alert('Thank you ' + response.getResponseText());
}