Apps Script Course: Variables
Before going any further, you need to understand some basic concepts such as variables and arrays which will be very useful to you later on.
Variables
A variable allows you to store a value (numeric, text, or other) so that it can be reused later in the code.
Before using a variable, you must start by declaring it with the let instruction followed by the name of the variable and a ; at the end of the line:
let variable;
For better readability of your code, choose names that describe the contents of the variable well. For example, for a variable that will contain the total annual cost, you can name it:
let totalAnnualCost;
For now, the variable totalAnnualCost has been declared but no value has been assigned to it.
To assign it the value 100, add a second line:
let totalAnnualCost;
totalAnnualCost = 100;
Or more simply, assign it this value during the declaration of the variable:
let totalAnnualCost = 100;
Types
A variable can store all sorts of values, for example numerical values:
let exampleValue1 = 123;
let exampleValue2 = 12.345;
let exampleValue3 = -54.3;
A variable can store text format values between '', "", or ``:
let exampleValue1 = 'Sheets-Pratique';
let exampleValue2 = "Course";
let exampleValue3 = `Apps Script`;
Note that if your text contains ' or ", the simplest is to surround the text with another character (or escape it with the \ character).
Example with the text "Apps Script" Course:
let exampleValue1 = '"Apps Script" Course';
let exampleValue2 = "\"Apps Script\" Course";
let exampleValue3 = `"Apps Script" Course`;
A variable can store boolean values true or false:
let exampleValue1 = true;
let exampleValue2 = false
A variable can also store more complex elements such as arrays, objects, functions, ..., which we will see later in this course.
Testing a Value
In this example, 3 variables are declared, the first is equal to 10, the second to 20, and the third to the sum of the first two:
let value1 = 10;
let value2 = 20;
let value3 = value1 + value2;
To test and verify the value of a variable, add:
console.log();
Then in parentheses, enter the value to be tested (here, that of the variable value3):
let value1 = 10;
let value2 = 20;
let value3 = value1 + value2;
console.log(value3);
And to execute this piece of code, open the document from the previous lesson (the one where you have already authorized macros), go to the editor and enter the code in the default function:
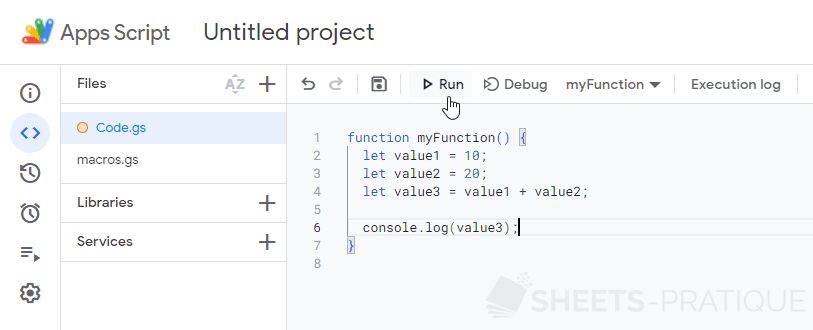
Then click on Run to execute the function.
You will then find in the execution log the value returned by console.log, 30:
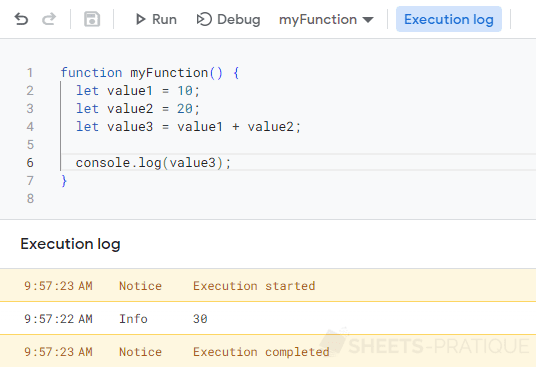
You can add several console.log if necessary:
let value1 = 10;
let value2 = 20;
let value3 = value1 + value2;
console.log(value1);
console.log(value2);
console.log(value3);
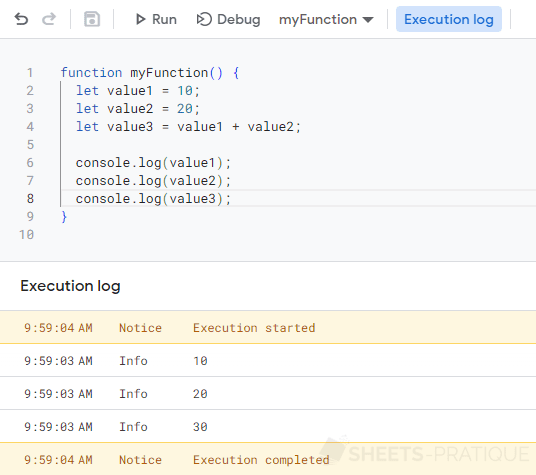