Create a MsgBox with Google Apps Script
Google Sheets allows you to create dialog boxes, choose which buttons to display, add a title, and retrieve the user's choice.
A simple example, often used in demonstrations, is:
Browser.msgBox('Example');
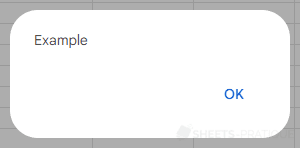
Choosing Buttons
To choose which buttons to display, add one of these values as a second argument:
- Browser.Buttons.OK: Ok button
- Browser.Buttons.OK_CANCEL: Ok, Cancel buttons
- Browser.Buttons.YES_NO: Yes, No buttons
- Browser.Buttons.YES_NO_CANCEL: Yes, No, Cancel buttons
For example:
function example() {
Browser.msgBox('Choose wisely!', Browser.Buttons.YES_NO_CANCEL);
}
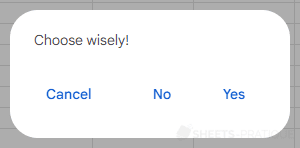
If you want to retrieve the user's choice, add a variable to receive this value:
function example() {
const choice = Browser.msgBox('Choose wisely!', Browser.Buttons.YES_NO_CANCEL);
}
The returned values (of type string) are:
- ok: clicking the Ok button
- yes: clicking the Yes button
- no: clicking the No button
- cancel: clicking the Cancel button (or no button)
Adding a Title
To add a title, add an additional argument containing the title, in the first position:
function example() {
Browser.msgBox('Your choice', 'Choose wisely!', Browser.Buttons.YES_NO_CANCEL);
}
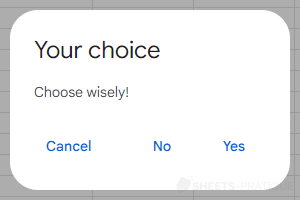
Request for Confirmation
Here is an example of a confirmation request:
function example() {
const choice = Browser.msgBox('Confirmation', 'Are you sure?', Browser.Buttons.YES_NO);
if (choice == 'yes') {
Browser.msgBox('You clicked "Yes"!');
}
}
Or:
function example() {
if (Browser.msgBox('Confirmation', 'Are you sure?', Browser.Buttons.YES_NO) == 'yes') {
Browser.msgBox('You clicked "Yes"!');
}
}
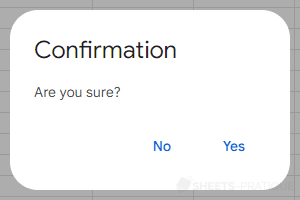
Alert Dialog Box
An alternative (recommended by Google) to using Browser.msgBox that also allows displaying dialog boxes is alert (more information on this method).