Custom Dialog Box with Apps Script
Google Sheets allows you to create your own dialog boxes using HTML, CSS, and JavaScript.
On this page, you will discover a simple example of a dialog box whose purpose is to display an image with a title and a button to close the window.
HTML Page
The window content is an HTML page, so start by adding an HTML file from the editor (which has been named logo in this example).
Default HTML tags are added.
Then insert the content of your dialog box (in HTML format) between the body tags:
<!DOCTYPE html>
<html>
<head>
</head>
<body>
<img src="https://www.sheets-pratique.com/template/img/en/logo.png">
<input type="button" value="Close" onclick="google.script.host.close()">
</body>
</html>
The following line corresponds to an image:
<img src="https://www.sheets-pratique.com/template/img/en/logo.png">
And this one to a button (with an action on click that closes the window):
<input type="button" value="Close" onclick="google.script.host.close()">
To add, for example, a paragraph, place your text between p tags:
<p>My paragraph ...</p>
Displaying the Dialog Box
The following function allows you to display the dialog box by integrating the HTML file named logo, with a width of 400 and a height of 132:
function example() {
const html = HtmlService.createHtmlOutputFromFile('logo')
.setWidth(400)
.setHeight(132);
SpreadsheetApp.getUi().showModalDialog(html, 'The website logo');
}
Preview:

Customizing the Style
Fortunately, you can modify the appearance of your HTML page content by adding CSS style rules between the head tags:
<!DOCTYPE html>
<html>
<head>
<style>
img {
display: block;
}
input {
display: inline-block;
float: right;
padding: 0.6rem 1rem 0.5rem;
margin-top: 1.5rem;
font-size: 1rem;
background: #dce3f9;
border: 2px solid #455fbb;
border-radius: 0.4rem;
cursor: pointer;
}
</style>
</head>
<body>
<img src="https://www.sheets-pratique.com/template/img/en/logo.png" alt="logo">
<input type="button" value="Close" onclick="google.script.host.close()">
</body>
</html>
Preview:
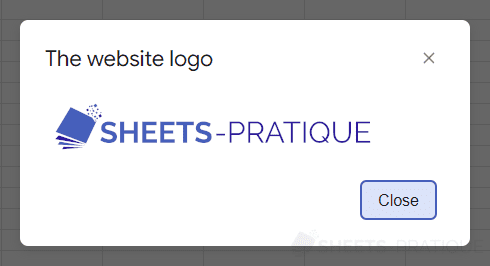
Another Example
An example of a more advanced custom dialog box is available on the page Create a Sidebar, here is a preview: